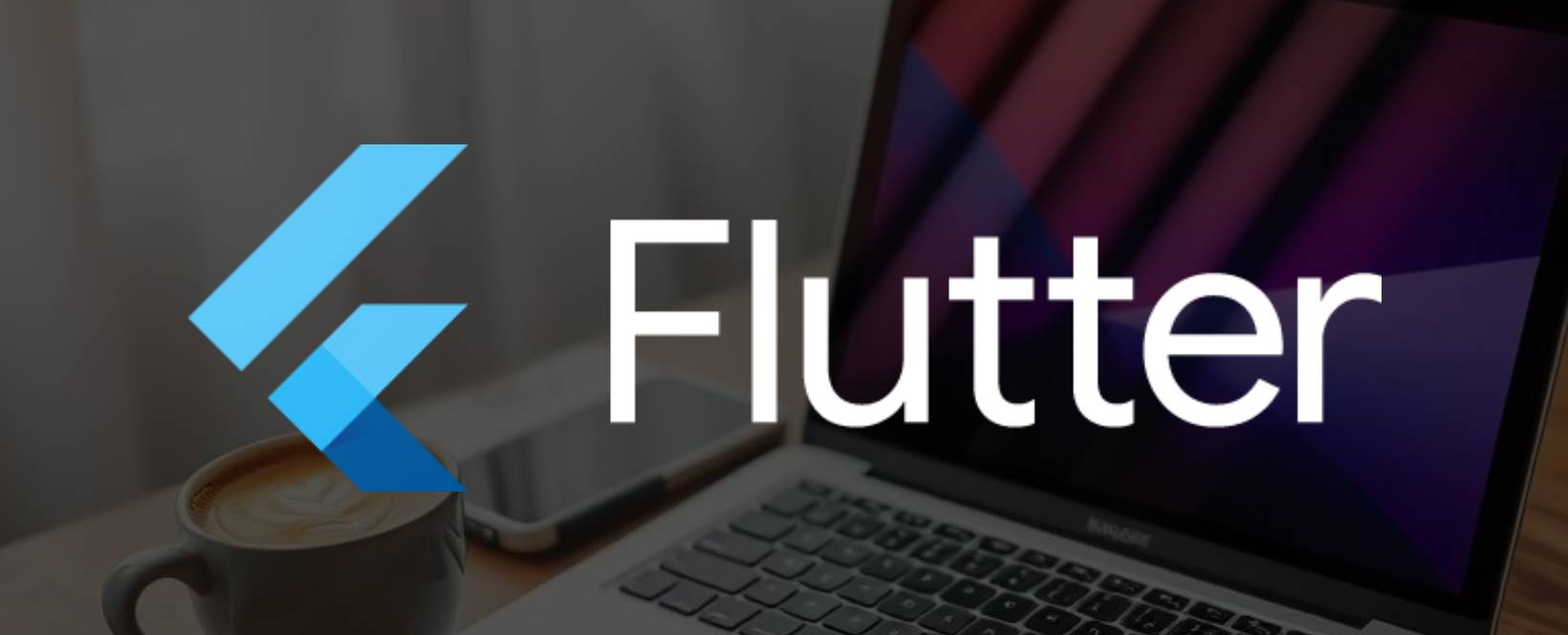
When working with APIs in Flutter, we often deal with JSON responses, which are represented as Map<String, dynamic> objects. However, accessing values from a map can lead to errors if a key is missing or has an unexpected type. To address this, we can create a MapParser extension that provides safe methods for parsing various data types.
In this tutorial, we’ll learn how to:
-
Create a MapParser extension.
-
Implement safe parsing methods for different data types.
-
Use the extension in a Flutter project to handle JSON responses effectively.
Step 1: Creating the MapParser Extension
Create a new Dart file (e.g., map_parser.dart) and add the following code:
Step 2: Using the MapParser Extension
To use this extension in your Flutter project, import map_parser.dart and apply it to JSON responses.
Example Usage
Let’s say we receive the following JSON response from an API:
{
"name": "John Doe",
"age": "30",
"salary": "45000.50",
"isActive": "true",
"details": {
"joinedDate": "2022-01-15T10:30:00Z"
}
}
We can safely parse this JSON using our MapParser extension:
import 'map_parser.dart';
void main() {
Map<String, dynamic> jsonResponse = {
"name": "John Doe",
"age": "30",
"salary": "45000.50",
"isActive": "true",
"details": {
"joinedDate": "2022-01-15T10:30:00Z"
}
};
String name = jsonResponse.parseStr("name");
int age = jsonResponse.parseInt("age");
double salary = jsonResponse.parseDouble("salary");
bool isActive = jsonResponse.parseBool("isActive");
DateTime? joinedDate = jsonResponse.parseMap("details").parseDateTime("joinedDate");
print("Name: $name");
print("Age: $age");
print("Salary: $salary");
print("Is Active: $isActive");
print("Joined Date: $joinedDate");
}
Expected Output:
Name: John Doe
Age: 30
Salary: 45000.5
Is Active: true
Joined Date: 2022-01-15 10:30:00.000Z
Conclusion
The MapParser extension makes it easier to handle JSON data in Flutter projects by providing safe parsing methods. With this approach:
-
We avoid null errors and type mismatches.
-
We can parse nested objects easily.
-
Our code becomes cleaner and more readable.
This extension is a simple yet powerful tool for working with APIs in Flutter. Try implementing it in your project to improve data parsing and error handling!